题目:给定一个排序数组和一个目标值,在数组中找到目标值,并返回其索引。如果目标值不存在于数组中,返回它将会被按顺序插入的位置。请必须使用时间复杂度为 O(log n)
的算法。
审题:
1、题目给定的是一个有序数组,对于有序数组的查找,首先应该想到二分法查找
2、题目要求,如果在目标值不存在的情况下,需要返回将要被插入的位置
3、题目要求,时间复杂度为O(log n)
,由此暴力查找的方式是行不通的
解析:
二分查找:二分法查找,也称作折半查找或对数查找,是一种在有序数组中查找某一特定元素的搜索算法。其基本原理是通过不断将待查找的区间分成两半,逐步缩小查找范围,直到找到目标元素或确定目标元素不存在。
二分法查找图解:
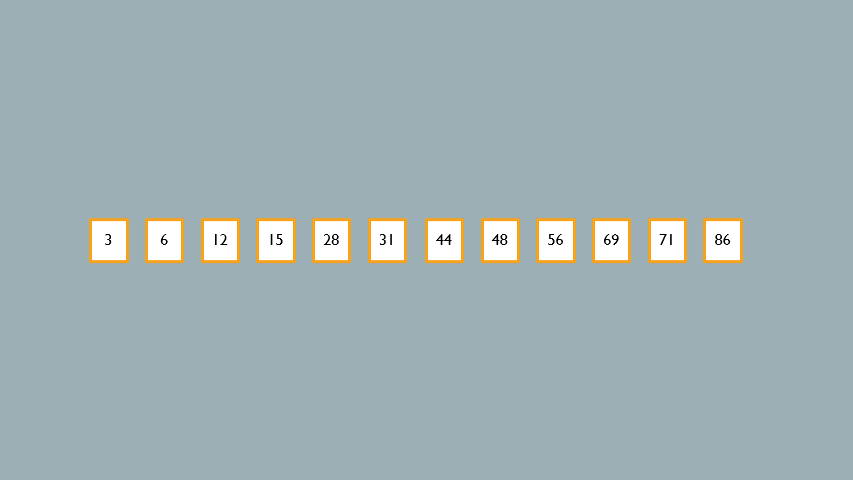
通过Java实现的该结题算法:
public class Solution {
public int searchInsert(int[] nums, int target) {
int left = 0;
int right = nums.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2; // 防止溢出
if (nums[mid] == target) {
return mid; // 找到目标值,返回索引
} else if (nums[mid] < target) {
left = mid + 1; // 目标值在右半部分
} else {
right = mid - 1; // 目标值在左半部分或不存在,缩小搜索范围
}
}
// 如果没有找到目标值,left就是插入位置
return left;
}
public static void main(String[] args) {
Solution solution = new Solution();
int[] nums = {1, 3, 5, 6};
int target = 5;
System.out.println(solution.searchInsert(nums, target)); // 输出: 2
target = 2;
System.out.println(solution.searchInsert(nums, target)); // 输出: 1
target = 7;
System.out.println(solution.searchInsert(nums, target)); // 输出: 4
target = 0;
System.out.println(solution.searchInsert(nums, target)); // 输出: 0
}
}
给你点赞
厉害厉害啊